Python is one of those languages that quietly becomes part of everything—from data crunching in research labs to automating tasks at home. It doesn’t try to overwhelm you. Instead, it waits for you to take the first step. And once you do, it’s surprisingly easy to follow. This guide breaks things down into concepts, helpful places to learn more, and project ideas to put your skills to use. So whether you’re completely new or brushing up after a break, there’s something here for you. Without any further ado, let’s have a look at it!
Core Concepts You Need to Understand
Variables and Data Types
Python lets you store information in a way that feels almost conversational. You don’t need to declare a variable’s type beforehand—just assign a value, and it understands what you mean. For example:
python name = "Alex" age = 25 is_student = True
Here, you’re storing a string, an integer, and a boolean—without saying much. That simplicity carries through the entire language.
Functions and Loops
Functions help you organize code so you’re not repeating the same instructions again and again. Here’s how you write one:
python def greet(name): return f"Hello, {name}!"
Loops come in when you want to repeat something multiple times. If you’ve got a list of names, for example, and want to greet each one, you’d write:
print(greet(name)) ```
Short and to the point. That’s the tone Python sticks to.
### Lists, Dictionaries, and Sets
These are the containers you’ll use most often. Lists keep things in order,
dictionaries match up keys with values, and sets make sure each item appears
only once.
```python my_list = [1, 2, 3] my_dict = {"name": "Sam", "age": 30} my_set =
{1, 2, 3} ```
Once you get the hang of these, everything else builds on top of them.
### Classes and Objects
When you want to model real-world things or structure larger programs, classes
step in. They act as blueprints. Let’s say you’re building a system for
tracking books:
```python class Book: def __init__(self, title, author): self.title = title
self.author = author ```
And then you can create as many books as you want using that same pattern:
```python book1 = Book("1984", "George Orwell") ```
You don’t need to know everything about object-oriented programming right
away, but understanding how to create and use a class will take you far.
## Resources That Actually Help
There’s no shortage of learning platforms, but not all of them are equally
helpful when you’re just getting started or trying to go beyond the basics.
Here are a few that get it right:
### 1\. Python Official Documentation
This is where you’ll find everything, straight from the source. It’s
structured, searchable, and surprisingly readable. When you’re confused about
syntax or want to double-check how a module works, this is the first place to
go.
### 2\. Real Python
Real Python balances detail with clarity. Their tutorials walk through things
step by step without assuming too much. Whether you’re looking into web
scraping, building command-line tools, or understanding decorators, this site
helps.
### 3\. Automate the Boring Stuff with Python
This one stands out for being practical. If your goal is to make life
easier—rename a hundred files, scrape a site, sort emails—this book shows how
to do it with clean, easy-to-follow examples.
### 4\. PyBites
This platform offers small, focused exercises that are perfect for sharpening
your skills. You can learn something meaningful even with just 15 minutes a
day.
### 5\. Stack Overflow
It might seem chaotic at first, but once you learn how to search effectively,
it becomes a goldmine of answers. Someone else has probably already hit the
same bug or design question you’re wrestling with.
## Projects Worth Building (No Matter Your Level)
You don’t really learn a language until you use it. That’s where projects come
in. Here are ideas that range from beginner-friendly to those that need a bit
more muscle.
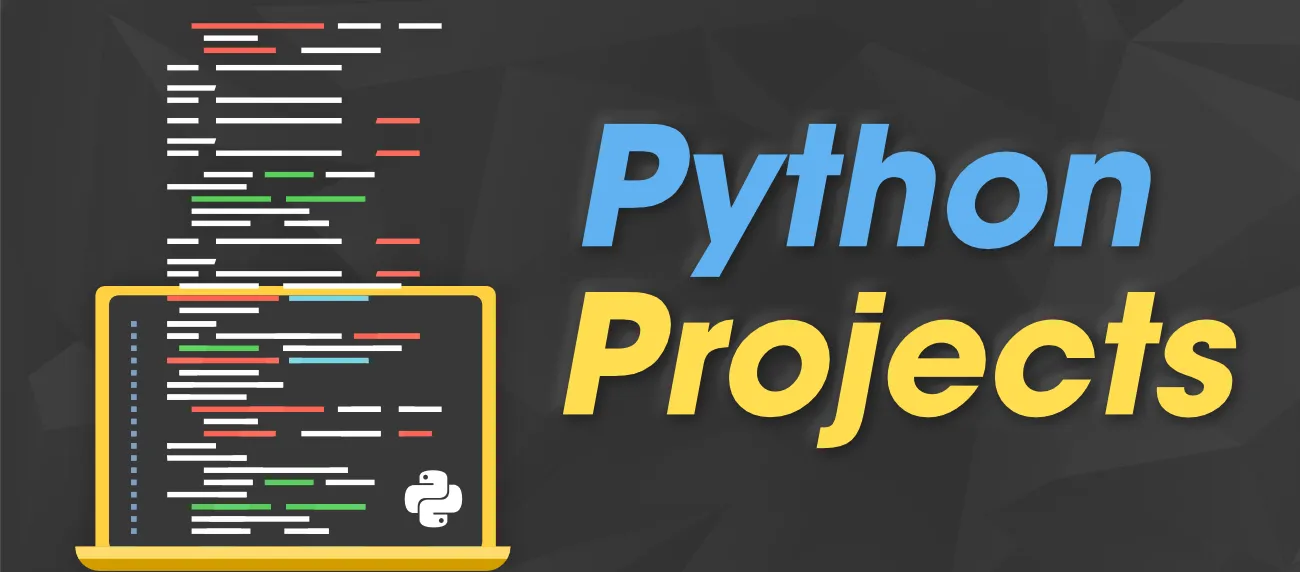
### 1\. Simple Budget Tracker
**What You’ll Use:**
* Input/output
* Lists and dictionaries
* File handling
Start with a script that takes in expenses and stores them in a CSV file. Add
categories like “food,” “rent,” or “subscriptions,” then calculate totals and
show trends. From there, you can move toward using libraries like pandas to
make the process cleaner.
### 2\. To-Do List with a GUI
**What You’ll Use:**
* Tkinter (for GUI)
* Functions and event handling
* File storage
This moves things off the command line. Create a small window where users can
type tasks, check them off, and clear completed ones. It’s a good intro to
working with buttons, input fields, and saving data.
### 3\. Web Scraper for Job Listings
**What You’ll Use:**
* requests and BeautifulSoup
* Loops and error handling
* Time module for scheduling
Write a script that pulls job titles, company names, and locations from a job
board. It can email you the results or save them in a spreadsheet. You’ll
learn a lot about how web pages are structured and how to pull only what you
need.
### 4\. Weather App Using an API
**What You’ll Use:**
* APIs
* JSON parsing
* GUI or command-line interface
Use a public weather API to pull real-time data based on city name. Then,
present the temperature, forecast, and maybe even suggestions like "Bring an
umbrella" or "You're good to go." It's a practical way to understand API calls
and how to format incoming data.
## Final Thoughts
Python’s biggest strength is how naturally it grows with you. You can use it
for tiny scripts that save time or full-scale systems that run websites,
analyze data, or power hardware. The key is to start somewhere—anywhere—and
let your curiosity shape the rest. Whether you’re drawn to automation, data,
apps, or games, there’s space for all of it here.