Humans rarely jump from question to answer in one shot. We naturally break complex problems into manageable pieces, connect ideas, and build understanding step by step. That same approach is gaining traction in AI through a technique called the “Skeleton of Thoughts.” It’s a modular reasoning method that guides models to structure their thinking into smaller, logical parts before expanding on them.
Instead of delivering one long response, the AI builds a scaffolded plan—much like an outline—then fills in each section. When implemented in Python, this method boosts clarity, traceability, and accuracy by reducing errors and promoting structured, self-correcting reasoning.
The Concept of Skeleton of Thoughts
Skeleton of Thoughts is a reasoning framework designed to improve how AI systems, particularly large language models, approach complex problems. Rather than delivering a continuous answer, this method divides the thinking process into two stages.
In the first stage, the AI generates a high-level outline—much like sketching the bones of a structure. Each “bone” represents a self-contained reasoning module, forming a scaffold or skeleton. Only after this framework is complete does the AI proceed to expand each point with detailed reasoning in the second stage.
This approach closely resembles how people write essays. You begin with an outline: define your thesis, list supporting points, and shape the conclusion. Only once the structure is clear do you start turning each point into paragraphs. With Skeleton of Thoughts, AI follows a similar rhythm—structure first, content second.
This two-pass thinking model has multiple benefits. It limits logical drift by keeping thoughts modular and localized. Since each reasoning step stands on its own, the model is less likely to lose track of its train of thought. It also allows for parallel processing, where each piece of the skeleton can be handled or reviewed independently. This modularity supports better feedback, easier revision, and even collective decision-making in multi-agent systems.
In applications like coding, scientific analysis, or multi-step math problems, the Skeleton of Thoughts adds a layer of clarity and discipline that pure freeform generation lacks. It offers more transparency and makes it easier to trace and improve individual reasoning steps.
Python Implementation of Skeleton of Thoughts
Let’s translate this idea into actual code. The goal is to simulate a reasoning process that first builds a skeleton and then populates it. We’ll use a language model API (like OpenAI’s GPT-4 or any alternative model) as the thinking engine. The Python Implementation will show how to structure prompts in two stages and how to handle modular thinking in practice.
First, define your task. Suppose the question is: “How can you improve traffic congestion in urban areas?”
Step 1: Generate the Skeleton
We prompt the model with the following:
into 4 main reasoning steps without solving it yet: {task}" response =
model.generate(prompt) return response ```
This might return something like:
* Identify primary causes of urban traffic.
* Analyze current mitigation strategies.
* Propose alternative innovative solutions.
* Evaluate the feasibility of implementation.
### Step 2: Fill in the Skeleton
Now that we have the structure, we can ask the model to expand each part:
```python def fill_skeleton(model, skeleton_steps): detailed_response = "" for
step in skeleton_steps: prompt = f"Expand on this reasoning step in detail:
{step}" response = model.generate(prompt) detailed_response +=
f"{step}\n{response}\n\n" return detailed_response ```
This method breaks reasoning into manageable, logical blocks, increasing
transparency. It also helps prevent the model from jumping to conclusions or
creating inconsistent arguments. Additionally, you can run these steps in
parallel threads or use voting to select the best expansion if you’re using
multiple models.
This Python Implementation showcases how AI systems can take advantage of
structured thinking. It reflects how a model can simulate a human-style
framework—think first, then elaborate—rather than trying to reason everything
in one breath.
## Benefits and Limitations in Practice
The biggest benefit of Skeleton of Thoughts is its control over complex
reasoning. You don’t just get an answer—you see how the answer was built. This
is especially helpful in sensitive applications like legal document drafting,
academic research, or financial analysis, where every assumption needs to be
visible.
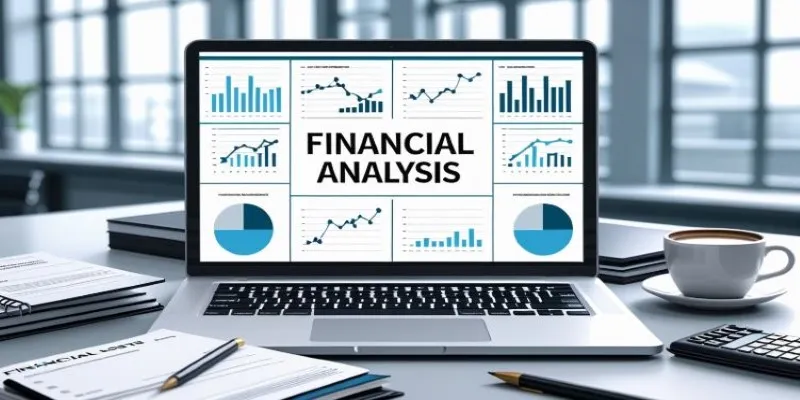
It also introduces modularity. If one part of the reasoning seems off, you can
regenerate just that section without affecting the rest. This is difficult to
do with standard, linear outputs from a language model.
From a coding perspective, the Python Implementation encourages better prompt
design. It also allows layering additional logic, such as review cycles and
feedback loops, and fine-tuning how detailed each expansion should be.
Moreover, it becomes easier to plug this structure into apps that need
explainability—like tutoring tools, medical reasoning bots, or multi-agent
decision-making systems.
Still, the method isn't perfect. One limitation is that it assumes the model
will stick to the skeleton it created. Sometimes, the expansions go off-track
or repeat content. Careful, prompt engineering is needed to ensure tight
coupling between structure and elaboration. It also relies heavily on prompt
clarity—vague skeleton steps will lead to vague expansions. Another challenge
is latency; breaking up a task into a skeleton and multiple detailed steps can
introduce time delays compared to a single-shot response.
But when precision matters more than speed, Skeleton of Thoughts is a powerful
choice.
## Conclusion
Skeleton of Thoughts offers a clearer way for AI to think—breaking down
problems into structured steps before diving into solutions. This two-stage
process mirrors how humans naturally reason and helps large language models
stay focused, reduce errors, and produce more logical outputs. When paired
with Python implementation, it becomes a practical tool for building smarter,
modular, and reviewable AI workflows. Whether you're designing a tutoring
system, analytical assistant, or research tool, this method adds transparency
and reliability. In a field where reasoning matters as much as results,
Skeleton of Thoughts sets the stage for more thoughtful AI behavior.